BBC's micro
Katarina, of whom we wrote about multiple times, actually has a lot of experience with teaching kids about programming and embedded development. So, she was happy to make a few examples for our new shield - micro
So, Katarina is going to lead you through all the necessary steps of making your own micro
Watch our video about the micro
Hardware requirements
Place the micro
Software requirements
The
Get set - ready - go
The idea behind this project is to implement a "distance reader" on the micro
The makecode editor itself offers the possibility of programming using blocks and using javascript editor. It will be necessary to create a custom module, for our application.
To make a new custom module:
- Go to JavaScript editor
- Click on the Explorer view and expand it
- Click on the + button that just appeared and add a custom.ts file in your project. Next, inside our custom.ts file we can add a function specifically for Proximity 2 click. Let’s start with the functions which are needed to initialize Proximity 2 click sensor.
Your custom.ts file should look like this.
namespace custom { //% block export function receive_config(): void { let buf = pins.createBuffer(2) buf.setNumber(NumberFormat.UInt8LE, 0, 0x02) buf.setNumber(NumberFormat.UInt8LE, 1, 0xF1) pins.i2cWriteBuffer(0x4A, buf, false) } //% block export function transmit_config(): void { let buf = pins.createBuffer(2) buf.setNumber(NumberFormat.UInt8LE, 0, 0x03) buf.setNumber(NumberFormat.UInt8LE, 1, 0x0F) pins.i2cWriteBuffer(0x4A, buf, false) } //% block export function main_config(): void { let buf = pins.createBuffer(2) buf.setNumber(NumberFormat.UInt8LE, 0, 0x01) buf.setNumber(NumberFormat.UInt8LE, 1, 0x13) pins.i2cWriteBuffer(0x4A, buf, false) } }
Let’s say that this is the default configuration for the Proximity 2 click. If you are interested what exactly each of these functions does, the datasheet for the MAX44000 is the place that offers answers.
Now we need to make the function which will read the distance from Proximity 2 click and play the tones on the Buzzer click, depending on the distance, but also power on the appropriate LEDs on the micro
You can add this function inside the custom.ts, below or above the previous ones.
//% block export function myTask(): void { let buf = pins.createBuffer(1) let distance = 0 let duration = 50 pins.analogSetPitchPin(AnalogPin.P0) buf.setNumber(NumberFormat.UInt8LE, 0, 0x16) pins.i2cWriteBuffer(0x4A, buf, false) buf = pins.i2cReadBuffer(0x4A, 1, false) distance = 255 - buf.getNumber(NumberFormat.UInt8LE, 0) if (distance < 20) { pins.analogPitch(3920, duration) basic.showLeds(`. . . . . . . . . . . . . . . . . . . . # # # # #`) } else if (distance > 20 && distance < 120) { pins.analogPitch(1760, duration) basic.showLeds(`. . . . . . . . . . . . . . . # # # # # . . . . .`) }
else if (distance > 120 && distance < 160) { pins.analogPitch(880, duration) basic.showLeds(`. . . . . . . . . . # # # # # . . . . . . . . . .`) } else if (distance > 160 && distance < 250) { pins.analogPitch(440, duration) basic.showLeds(`. . . . . # # # # # . . . . . . . . . . . . . . .`) } else if (distance > 250) { pins.analogPitch(110, duration) basic.showLeds(`# # # # # . . . . . . . . . . . . . . . . . . . .`) } }
This function actually reads the distance value from the 0x16 register using I2C communication and checks if the distance is in any of the offered range. If the distance is smaller the tone frequency will be higher. We used the PWM frequency to adjust the sound frequency.
These four functions will be enough for us to finish the demo using the
Now let’s add the “configuration” functions to the “on start” container and our task function to the “forever” container.
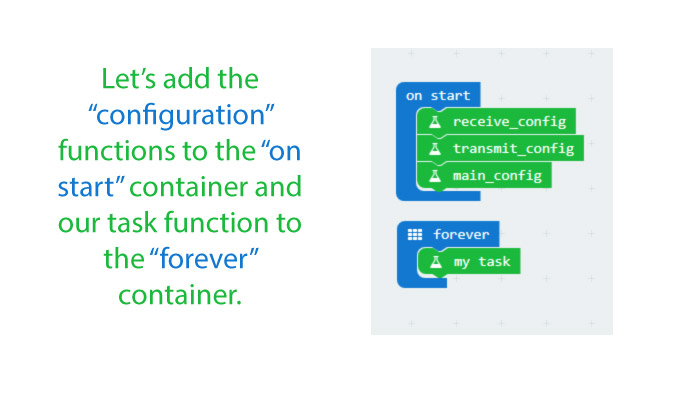
That’s it, we can now click on the download button and upload the hex file to our micro
The uploading is nothing more than the copying of the file inside
Conclusion
Now it's time for you to go and go try it out for yourself. "Trial and error" is one of the best ways to learn, so don't get discouraged if you don't succeed on your first try.
With a detailed tutorial like this one, all you need is some practice.