3.5 Timer TMR1
Timer TMR1 module is a 16-bit timer/counter, which means that it consists of two registers (TMR1L and TMR1H). It can count up 65.535 pulses in a single cycle, i.e. before the counting starts from zero.
Similar to the timer TMR0, these registers can be read or written to at any moment. In case an overflow occurs, an interrupt is generated if enabled.
The timer TMR1 module may operate in one of two basic modes, that is as a timer or a counter. Unlike the TMR0 timer, both of these modes have additional functions.
The TMR1 timer has following features:
- 16-bit timer/counter register pair;
- Programmable internal or external clock source;
- 3-bit prescaler;
- Optional LP oscillator;
- Synchronous or asynchronous operation;
- Timer TMR1 gate control (count enable) via comparator or T1G pin;
- Interrupt on overflow;
- Wake-up on overflow (external clock); and
- Time base for Capture/Compare function.
TIMER TMR1 CLOCK SOURCE SELECTION
The TMR1CS bit of the T1CON register is used to select the clock source for this timer:
CLOCK SOURCE |
TMR1CS |
Fosc/4 |
0 |
T1CKI pin |
1 |
When the internal clock source is selected, the TMR1H-TMR1L register pair will be incremented on multiples of Fosc pulses as determined by the prescaler.
When the external clock source is selected, this timer may operate as a timer or a counter. Clock in counter mode can be synchronized with the microcontroller internal clock or run asynchronously. In the event that an external clock oscillator is needed and the PIC16F887 microcontroller is using INTOSC with CLKOUT, timer TMR1 can use the LP oscillator as a clock source.
TIMER TMR1 PRESCALER
Timer TMR1 has a completely separate prescaler which allows 1, 2, 4 or 8 division of the clock input frequency. The prescaler is not directly readable or writable. However, the prescaler counter is automatically cleared after writing to the TMR1H or TMR1L register.
TIMER TMR1 OSCILLATOR
RC0/T1OSO and RC1/T1OSI pins are used to register pulses coming from peripheral electronics, but they also have an additional function. As seen in figure, they are simultaneously configured as both input (pin RC1) and output (pin RC0) of additional LP quartz oscillator (
Low Power). This circuit is primarily designed for the operation at low frequencies (up to 200 KHz), more precisely, for the use of 32,768 KHz quartz crystal. Such crystals are used in quartz watches because it is easy to obtain one-second-long pulses by dividing this frequency.
Since this oscillator does not depend on internal clock, it can operate even in
sleep mode. It is enabled by setting the T1OSCEN control bit of the T1CON register. The user must provide a software time delay (a few milliseconds) to enable the oscillator to start up properly.
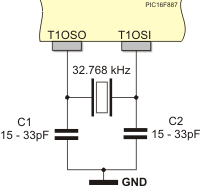
Table below shows the recommended values of the capacitors to suit the quartz oscillator. These values do not have to be exact. However, the general rule is: the higher the capacity, the higher the stability, which, at the same time, prolongs the time needed for oscillator stability.
OSCILLATOR |
FREQUENCY |
C1 |
C2 |
LP |
32 kHz |
33 pF |
33 pF |
100 kHz |
15 pF |
15 pF |
200 kHz |
15 pF |
15 pF |
TIMER TMR1 GATE
Timer TMR1 gate source is software configurable to be the T1G pin or the output of comparator C2. This gate allows the timer to directly time external events using the logic state of the T1G pin or analog events using the comparator C2 output. Refer to figure on the previous page. In order to measure a signal duration, it is sufficient to enable this gate and count pulses passing through it.
THE USE OF TIMER TMR1 OSCILLATOR
The power consumption of the microcontroller is reduced to the lowest level in Sleep mode since the main power consumer - the oscillator - doesn’t operate. It is easy to set the microcontroller in this mode- by executing the
SLEEP instruction. The problem is how to wake up the microcontroller because only an interrupt can make it happen. Since the microcontroller 'sleeps', an interrupt must be triggered by external electronics. It gets incredibly complicated if it is necessary to wake up the microcontroller at regular time intervals...
In order to solve this problem, a completely independent
Low Power quartz oscillator, capable of operating in
sleep mode, is built into the PIC16F887 microcontroller. Simply put, a previously separate circuit is now built into the microcontroller and assigned to the timer TMR1. The oscillator is enabled by setting the T1OSCEN bit of the T1CON register. The TMR1CS bit of the same register is then used to enable the timer TMR1 to use pulse sequences from that oscillator.
- A signal generated by this quartz oscillator is synchronized with the microcontroller clock by clearing the T1SYNC bit. In this case, the timer cannot operate in sleep mode because the circuit for synchronization uses the microcontroller clock.
- The TMR1 register overflow interrupt can be enabled. If the T1SYNC bit is set, such interrupts will also occur in sleepmode.
TMR1 IN TIMER MODE
In order to select this mode, it is necessary to clear the TMR1CS bit. After this, the 16-bit register will be incremented on every pulse generated by the internal oscillator. If the 4MHz quartz crystal is in use, it will be incremented every microsecond.
In this mode, the T1SYNC bit does not affect the timer because it counts internal clock pulses. Since the whole electronics uses these pulses, there is no need for synchronization.
The microcontroller’s clock oscillator does not operate during
sleep mode so the timer register overflow cannot cause any interrupt.
Let's do it in mikroC...
// In this example, TMR1 is configured as a timer with the prescaler rate 1:8. Every time
// TMR1H and TMR1L registers overflow occurs, an interrupt will be requested.
void main() {
PIR1.TMR1IF = 0; // Reset the TMR1IF flag bit
TMR1H = 0x22; // Set initial value for the timer TMR1
TMR1L = 0x00;
TMR1CS = 0; // Timer1 counts pulses from internal oscillator
T1CKPS1 = T1CKPS0 = 1; // Assigned prescaler rate is 1:8
PIE1.TMR1IE = 1; // Enable interrupt on overflow
INTCON = 0xC0; // Enable interrupt (bits GIE and PEIE)
TMR1ON = 1; // Turn the timer TMR1 on
...
TMR1 IN COUNTER MODE
Timer TMR1 starts to operate as a counter by setting the TMR1CS bit. It counts pulses brought to the PC0/T1CKI pin and is incremented on the rising edge of the external clock input T1CKI. If the control bit T1SYNC of the T1CON register is cleared, the external clock inputs will be synchronized on their way to the TMR1 register. In other words, the timer TMR1 is synchronized to the microcontroller system clock and is called a synchronous counter.
When the microcontroller, operating in this way, is set in
sleep mode, the TMR1H and TMR1L timer registers are not incremented even though clock pulses appear on the input pins. Since the microcontroller system clock doesn’t run in this mode, there are no clock inputs to be used for synchronization. However, the prescaler will continue to run as far as there are clock pulses on the pins because it is just a simple frequency divider.
This counter registers a logic one (1) on input pins. It is important to know that at least one falling edge must be registered prior to starting pulse counting. Refer to figure on the left. The arrows in figure denote counter increments.
T1CON Register
T1GINV - Timer1 Gate Invert bit acts as logic state inverter on the T1G pin gate or the comparator C2 output (C2OUT) gate. It enables the timer to mea sure time whilst the gate is high or low.
- 1 - Timer 1 counts when the T1G pin or bit C2OUT gate is high (1).
- 0 - Timer 1 counts when the T1G pin or bit C2OUT gate is low (0).
TMR1GE - Timer1 Gate Enable bit determines whether the T1G pin or comparator C2 output (C2OUT) gate will be active or not. This bit is functional only in the event that the timer TMR1 is on (bit TMR1ON = 1). Otherwise, this bit is ignored.
- 1 - Timer TMR1 is on only if Timer1 gate is not active.
- 0 - Gate has no influence on the timer TMR1.
T1CKPS1, T1CKPS0 - Determine the rate of the prescaler assigned to the timer TMR1.
T1CKPS1 |
T1CKPS0 |
PRESCALER RATE |
0 |
0 |
1:1 |
0 |
1 |
1:2 |
1 |
0 |
1:4 |
1 |
1 |
1:8 |
T1OSCEN - LP Oscillator Enable Control bit
- 1 - LP oscillator is enabled for timer TMR1 clock (oscillator with low power consumption and frequency 32.768 kHz).
- 0 - LP oscillator is off.
T1SYNC - Timer1 External Clock Input Synchronization Control bit enables synchronization of the LP oscillator input or T1CKI pin input with the microcontroller internal clock. This bit is ignored while counting pulses from the main oscillator (bit TMR1CS = 0).
- 1 - Do not synchronize external clock input.
- 0 - Synchronize external clock input.
TMR1CS - Timer TMR1 Clock Source Select bit
- 1 - Count pulses on the T1CKI pin (on the rising edge 0-1).
- 0 - Count pulses of the microcontroller internal clock.
TMR1ON - Timer1 On bit
- 1 - Enable timer TMR1.
- 0 - Stop timer TMR1.
In Short
In order to use the timer TMR1 properly, it is necessary to perform the following:
- Since it is not possible to turn off the prescaler, its rate should be adjusted by using bits T1CKPS1 and T1CKPS0 of the register T1CON (Refer to table).
- Select the mode by the TMR1CS bit of the same register (TMR1CS: 0=the clock source is quartz oscillator, 1= the clock source is supplied externally).
- By setting the T1OSCEN bit of the same register, the oscillator is enabled and the TMR1H and TMR1L registers are incremented on every clock input. Counting stops by clearing this bit.
- The prescaler is cleared by clearing or writing to the counter registers.
- By filling both timer registers, the flag TMR1IF is set and counting starts from zero.