4.16 Example 14
Using graphic LCD display
A graphic LCD (GLCD) provides an advanced method for displaying visual messages. While the character LCD can display only alphanumeric characters, the GLCD can display messages in the form of drawings and bitmaps. The most commonly used graphic LCD has 128x64 pixels screen resolution. The GLCD contrast can be adjusted using the potentiometer P1.
Here, the GLCD displays a picture of truck the bitmap of which is stored in the
truck_bmp.c
file.
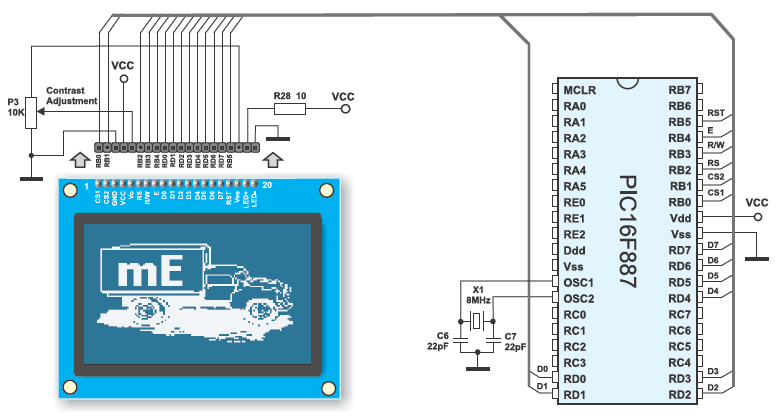
/*Header******************************************************/
//Declarations------------------------------------------------------------------
const code char truck_bmp[1024];
//--------------------------------------------------------------end-declarations
// Glcd module connections
char GLCD_DataPort at PORTD;
sbit GLCD_CS1 at RB0_bit;
sbit GLCD_CS2 at RB1_bit;
sbit GLCD_RS at RB2_bit;
sbit GLCD_RW at RB3_bit;
sbit GLCD_EN at RB4_bit;
sbit GLCD_RST at RB5_bit;
sbit GLCD_CS1_Direction at TRISB0_bit;
sbit GLCD_CS2_Direction at TRISB1_bit;
sbit GLCD_RS_Direction at TRISB2_bit;
sbit GLCD_RW_Direction at TRISB3_bit;
sbit GLCD_EN_Direction at TRISB4_bit;
sbit GLCD_RST_Direction at TRISB5_bit;
// End Glcd module connections
void delay2S(){ // 2 second delay function
Delay_ms(2000);
}
void main() {
unsigned short ii;
char *someText;
#define COMPLETE_EXAMPLE
ANSEL = 0; // Configure AN pins as digital
ANSELH = 0;
C1ON_bit = 0; // Disable comparators
C2ON_bit = 0;
Glcd_Init(); // Initialize GLCD
Glcd_Fill(0x00); // Clear GLCD
while(1) {
#ifdef COMPLETE_EXAMPLE
Glcd_Image(truck_bmp); // Draw image
delay2S(); delay2S();
#endif
Glcd_Fill(0x00); // Clear GLCD
Glcd_Box(62,40,124,56,1); // Draw box
Glcd_Rectangle(5,5,84,35,1); // Draw rectangle
Glcd_Line(0, 0, 127, 63, 1); // Draw line
delay2S();
for(ii = 5; ii < 60; ii+=5 ){ // Draw horizontal and vertical lines
Delay_ms(250);
Glcd_V_Line(2, 54, ii, 1);
Glcd_H_Line(2, 120, ii, 1);
}
delay2S();
Glcd_Fill(0x00); // Clear GLCD
#ifdef COMPLETE_EXAMPLE
Glcd_Set_Font(Character8x7, 8, 7, 32); // Choose font, see __Lib_GLCDFonts.c
// in Uses folder
#endif
Glcd_Write_Text("mikroE", 1, 7, 2); // Write string
for (ii = 1; ii <= 10; ii++) // Draw circles
Glcd_Circle(63,32, 3*ii, 1);
delay2S();
Glcd_Box(12,20, 70,57, 2); // Draw box
delay2S();
#ifdef COMPLETE_EXAMPLE
Glcd_Fill(0xFF); // Fill GLCD
Glcd_Set_Font(Character8x7, 8, 7, 32); // Change font
someText = "8x7 Font";
Glcd_Write_Text(someText, 5, 0, 2); // Write string
delay2S();
Glcd_Set_Font(System3x5, 3, 5, 32); // Change font
someText = "3X5 CAPITALS ONLY";
Glcd_Write_Text(someText, 60, 2, 2); // Write string
delay2S();
Glcd_Set_Font(font5x7, 5, 7, 32); // Change font
someText = "5x7 Font";
Glcd_Write_Text(someText, 5, 4, 2); // Write string
delay2S();
Glcd_Set_Font(FontSystem5x7_v2, 5, 7, 32); // Change font
someText = "5x7 Font (v2)";
Glcd_Write_Text(someText, 5, 6, 2); // Write string
delay2S();
#endif
}
}
truck_bmp.c file:
// ------------------------------------------------------
// GLCD Picture name: truck.bmp
// GLCD Model: KS0108 128x64
// ------------------------------------------------------
unsigned char const truck_bmp[1024] = {
0, 0, 0, 0, 0,248, 8, 8, 8, 8, 8, 8, 12, 12, 12, 12,
12, 10, 10, 10, 10, 10, 10, 9, 9, 9, 9, 9, 9, 9, 9, 9,
9, 9, 9, 9, 9, 9, 9, 9, 9, 9,137,137,137,137,137,137,
137,137,137,137,137,137,137, 9, 9, 9, 9, 9, 9, 9, 9, 9,
9, 9, 13,253, 13,195, 6,252, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0,255, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
240,240,240,240,240,224,224,240,240,240,240,240,224,192,192,224,
240,240,240,240,240,224,192, 0, 0, 0,255,255,255,255,255,195,
195,195,195,195,195,195, 3, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0,255,240, 79,224,255, 96, 96, 96, 32, 32, 32, 32, 32,
32, 32, 32, 32, 32, 32, 32, 32, 64, 64, 64, 64,128, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0,255, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
255,255,255,255,255, 0, 0, 0, 0,255,255,255,255,255, 0, 0,
0, 0,255,255,255,255,255, 0, 0, 0,255,255,255,255,255,129,
129,129,129,129,129,129,128, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0,255, 1,248, 8, 8, 8, 8, 8, 8, 8, 8, 8, 8,
8, 8, 8, 8, 16,224, 24, 36,196, 70,130,130,133,217,102,112,
160,192, 96, 96, 32, 32,160,160,224,224,192, 64, 64,128,128,192,
64,128, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 63, 96, 96, 96,224, 96, 96, 96, 96, 96, 96,
99, 99, 99, 99, 99, 96, 96, 96, 96, 99, 99, 99, 99, 99, 96, 96,
96, 96, 99, 99, 99, 99, 99, 96, 96, 96, 99, 99, 99, 99, 99, 99,
99, 99, 99, 99, 99, 99, 99, 96, 96, 96, 96, 96, 96, 96, 64, 64,
64,224,224,255,246, 1, 14, 6, 6, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2,130, 67,114, 62, 35, 16, 16, 0, 7, 3, 3, 2,
4, 4, 4, 4, 4, 4, 4, 28, 16, 16, 16, 17, 17, 9, 9, 41,
112, 32, 67, 5,240,126,174,128, 56, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1,
1, 1,127,127,127,127,255,255,247,251,123,191, 95, 93,125,189,
189, 63, 93, 89,177,115,243,229,207, 27, 63,119,255,207,191,255,
255,255,255,255,255,255,255,127,127,127,127,127,127,127,127,255,
255,255,127,127,125,120,120,120,120,120,248,120,120,120,120,120,
120,248,248,232,143, 0, 0, 0, 0, 0, 0, 0, 0,128,240,248,
120,188,220, 92,252, 28, 28, 60, 92, 92, 60,120,248,248, 96,192,
143,168,216,136, 49, 68, 72, 50,160, 96, 0, 0, 0, 0, 0, 0,
0, 0, 0,128,192,248,248,248,248,252,254,254,254,254,254,254,
254,254,254,254,254,255,255,255,255,255,246,239,208,246,174,173,
169,128,209,208,224,247,249,255,255,252,220,240,127,255,223,255,
255,255,255,255,255,254,254,255,255,255,255,255,255,255,254,255,
255,255,255,255,255,255,254,254,254,254,254,254,254,254,254,254,
254,254,254,254,255,255,255,255,255,255,254,255,190,255,255,253,
240,239,221,223,254,168,136,170,196,208,228,230,248,127,126,156,
223,226,242,242,242,242,242,177, 32, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 1, 1, 1, 1, 3, 3, 3, 7, 7, 7, 7, 7, 15,
15, 15, 7, 15, 15, 15, 7, 7, 15, 14, 15, 13, 15, 47, 43, 43,
43, 43, 43, 47,111,239,255,253,253,255,254,255,255,255,255,255,
191,191,239,239,239,191,255,191,255,255,255,255,255,255,255,255,
255,255,255,255,255,255,255,255,255,255,255,255,255,255,255,255,
255,255,255,255,127,127,127,127,255,255,191,191,191,191,255,254,
255,253,255,255,255,251,255,255,255,127,125, 63, 31, 31, 31, 31,
31, 31, 63, 15, 15, 7, 7, 3, 3, 3, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0,
1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1,
1, 1, 1, 1, 3, 3, 3, 11, 11, 11, 11, 7, 3, 14, 6, 6,
6, 2, 18, 19, 19, 3, 23, 21, 21, 17, 1, 19, 19, 3, 6, 6,
14, 15, 15, 7, 15, 15, 15, 11, 2, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
};
In order to make this example work properly, it is necessary to tick off the GLCD library in the
Library Manager prior to compiling. Also, it is necessary to include document
truck_bmp.c into the project.