3.5 Timer1
Timer1 module is a 16-bit timer/counter, which means that it consists of two registers (TMR1L and TMR1H). It is capable of counting up to 65.535 pulses in a single cycle, i.e. before the counting starts from zero.
Similar to Timer0, these registers can be read or written to at any point. In case an overflow occurs, an interrupt is automatically generated, provided it is enabled.
The Timer1 may be set to operate in one of two modes, either as a timer or a counter. Unlike the Timer0, both of these modes give additional possibilitis.
The Timer1 has the following features:
- 16-bit timer/counter register pair;
- Programmable internal or external clock source;
- 3-bit prescaler;
- Optional Low Power (LP) oscillator;
- Synchronous or asynchronous operation;
- Timer1 gate control (count enabled) via comparator or the T1G pin;
- Interrupt on overflow;
- Wake-up on overflow (external clock); and
- Clock source for Capture/Compare module.
A simplified schematic of the Timer1
TIMER TMR1 CLOCK SOURCE SELECTION
The TMR1CS bit of the T1CON register is used for selecting the clock source for the Timer1:
CLOCK SOURCE |
TMR1CS |
Fosc/4 |
0 |
T1CKI pin |
1 |
When the internal clock source is selected, the TMR1H-TMR1L register pair will be incremented on multiples of Fosc pulses as determined by the prescaler.
When the external clock source is selected, the timer may operate either as a timer or a counter. Clock pulses in the counter mode can be synchronized with the microcontroller internal clock or run asynchronously.
TIMER1's PRESCALER
Timer1 is provided with a completely independent prescaler which allows 1, 2, 4 or 8 division of the clock input frequency. The prescaler is not directly readable or writable. However, the prescaler counter is automatically cleared after write to the TMR1H or TMR1L register.
TIMER1's OSCILLATOR
The RC0/T1OSO and RC1/T1OSI pins are, above all, used to register pulses coming from peripheral modules, but they also have an additional function. As can be seen in figure, they simultaneously act as input (pin RC1) and output (pin RC0) of additional LP quartz oscillator (Low Power). This circuit is primarily designed for the operation at low frequencies (up to 200 KHz) and uses 32,768 KHz quartz crystal. Such crystal is convenient for quartz watches because it is easy to obtain one-second-long pulses by dividing this frequency. Since the operation of this oscillator does not depend on the internal clock, it is capable of operating even in sleep mode, which is enabled by setting the T1OSCEN control bit of the T1CON register. In this case it is necessary to provide a short time delay (a few milliseconds) from within the software for the sake of the clock oscillator stability.
Table below contains the recommended values of the capacitors which are, in addition to quartz crystal, integrated into the oscillator. These values are not critical and the following rule applies: the higher the capacity, the higher the stability, which, at the same time, prolongs the time necessary for the clock oscillator stability.
OSCILLATOR |
FREQUENCY |
C1 |
C2 |
LP |
32 kHz |
33 pF |
33 pF |
100 kHz |
15 pF |
15 pF |
200 kHz |
15 pF |
15 pF |
The power consumption of the microcontroller is minimized in the Sleep mode as the main power consumer, the oscillator, doesn’t operate. The microcontroller is set to this mode by executing the SLEEP instruction. The problem is how to wake up the microcontroller, i.e. how to generate an interrupt which makes it happen. Since the microcontroller ‘sleeps’, an interrupt must be triggered by peripheral modules. It gets even more complicated if it is necessary to wake up the microcontroller at regular time intervals...
The problem is solved by embedding a completely independent Low Power quartz oscillator capable of operating in sleep mode into the PIC16F887 microcontroller. Simply put, the module that used to be peripheral is now built into the microcontroller and assigned to the Timer1. The oscillator is enabled by setting the T1OSCEN bit of the T1CON register. The TMR1CS bit is then used to enable the Timer1 to use pulses generated by that oscillator.
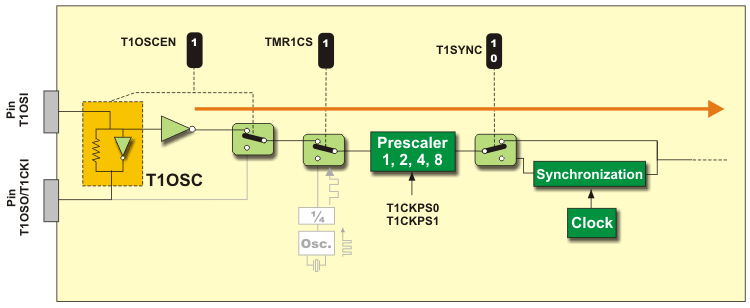
- A signal generated by the quartz oscillator is synchronized with the microcontroller clock by clearing the T1SYNC bit. In this case, the timer cannot operate in sleep mode because the circuit for synchronization uses the microcontroller clock.
- The TMR1 register overflow interrupt can be enabled. If the T1SYNC bit is set, such interrupts will also occur in sleep mode.
TIMER1'S GATE
The Timer1 gate source is software configurable to use the T1G pin or the output of comparator C2. This gate allows the timer to directly time external signals using the T1G pin or analog signals using the comparator C2 output. Refer to figure on the previous page. In order to measure a signal duration, it is sufficient to enable this gate and count pulses in the TMR1 register.
TIMER1 IN COUNTER MODE
Timer1 starts to operate as a counter by setting the TMR1CS bit. It counts pulses supplied to the PC0/T1CKI pin and is incremented on the pulse rising edge on the external clock input T1CKI. If the control bit T1SYNC of the T1CON register is cleared, the external clock inputs will be synchronized before they reach the TMR1 register pair. In other words, the Timer1 is synchronized with the microcontroller clock, thus becoming a synchronous counter.
If the microcontroller is set to sleep mode while the Timer1 operates as a counter, the TMR1H and TMR1L timer registers will not be modified even though the input pins are fed with pulses. Since the microcontroller clock doesn’t run in sleep mode, there are no clock inputs to be used for synchronization. However, the prescaler will keep on running as far as there are clock pulses on the pins because it is just a simple frequency divider.
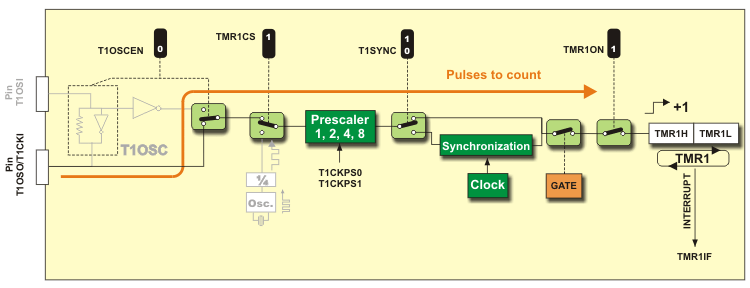
This counter registers a logic one (1) on input pins. The thing is that at least one falling edge must be registered prior to starting pulse counting. Refer to figure on the left. The arrows denote counter increments.
TIMER1 IN TIMER MODE
In order to set the Timer1 to timer mode, it is necessary to clear the TMR1CS bit. After that, every pulse generated by the internal oscillator will cause the 16-bit timer register to be incremented. If the 4MHz quartz crystal is in use, this register will be incremented every microsecond.
In this mode, the T1SYNC bit does not affect the timer because it counts internal clock pulses used by the microcontroller and other internal modules. Hence there is no need for synchronization.
The microcontroller clock does not operate in sleep mode so that the timer register overflow cannot cause an interrupt in this mode.
Let's do it in mikroBasic...
' In this example, Timer1 is configured as a timer with the prescaler rate 1:8. Every time
' TMR1H and TMR1L registers overflow occurs, an interrupt will be requested.
main:
...
PIR1.TMR1IF = 0 ' Reset the TMR1IF flag bit
TMR1H = 0x22 ' Set initial value for the Timer1
TMR1L = 0x00
T1CON.TMR1CS = 0 ' Timer1 counts pulses from internal oscillator
T1CON.T1CKPS0 = 1 ' Assigned prescaler rate is 1:8
T1CON.T1CKPS1 = 1
PIE1.TMR1IE = 1 ' Enable interrupt on overflow
INTCON = 0xC0 ' Enable interrupt (bits GIE and PEIE)
T1CON.TMR1ON = 1 ' Turn the Timer1 on
...
T1CON Register
Bits of the T1CON register are in control of the Timer1 operation.
T1GINV - Timer1 Gate Invert bit acts as a logic state inverter on the T1G pin gate or the comparator C2 output (C2OUT) gate. It enables the timer to count pulses while the gate is high or low.
- 1 - Timer 1 counts when the T1G pin or bit C2OUT gate is high (1).
- 0 - Timer 1 counts when the T1G pin or bit C2OUT gate is low (0).
TMR1GE - Timer1 Gate Enable bit determines whether the T1G pin or the com parator C2 output (C2OUT) gate will be active or not. This bit is enabled only when the Timer1 is on (bit TMR1ON = 1). Otherwise, it is ignored.
- 1 - Timer1 is enabled only when the Timer1 gate is not active.
- 0 - Gate doesn’t affect the operation of Timer1.
T1CKPS1, T1CKPS0 - determine the rate of the prescaler assigned to the Timer1.
T1CKPS1 |
T1CKPS0 |
PRESCALER RATE |
0 |
0 |
1:1 |
0 |
1 |
1:2 |
1 |
0 |
1:4 |
1 |
1 |
1:8 |
T1OSCEN - LP Oscillator Enable Control bit
- 1 - LP oscillator is enabled for the Timer1 clock (a low-power oscillator with a frequency of 32.768 kHz).
- 0 - LP oscillator is disabled.
T1SYNC - Timer1 External Clock Input Synchronization Control bit enables the LP oscillator input or the T1CKI pin input to be synchronized with the micro controller internal clock. The bit is ignored as long as pulses supplied from the microcontroller clock source are counted (bit TMR1CS = 0).
- 1 - External clock input will not be synchronized..
- 0 - External clock input will be synchronized..
TMR1CS - Timer TMR1 Clock Source Select bit
- 1 - Count pulses on the T1CKI pin (on the rising edge 0-1).
- 0 - Count pulses generated by the microcontroller clock.
TMR1ON - Timer1 On bit
- 1 - Timer1 enabled.
- 0 - Timer1 disabled.
In Short
In order to use the Timer1 properly, it is necessary to do the following:
- Since it is not possible to turn off the prescaler, its rate should be adjusted by using bits T1CKPS1 and T1CKPS0 of the register T1CON (Refer to the previous table).
- Select the appropriate mode using the TMR1CS bit of the register T1CON. (TMR1CS: 0=the clock source is internal quartz oscillator, 1= the clock is supplied externally).
- By setting the T1OSCEN bit of the same register, the oscillator is enabled and the TMR1H and TMR1L registers are incremented on every clock pulse. Counting stops by clearing this bit.
- The prescaler is cleared by clearing or writing to the counter registers.
- When both timer registers are filled with ones, an overflow occures, the TMR1IF flag is set and counting starts from zero.