3.2 Core SFRs
The following text describes the core SFRs of the PIC16F887 microcontroller. Their bits control different modules within the chip and therefore are described along with processes they are in control of.
STATUS Register
The STATUS register contains the arithmetic status of data in the W register, reset status and bank select bits for data memory.
- IRP - Bit is used for selecting register bank at indirect addressing.
- 1 - Banks 0 and 1 are active (memory locations 00h-FFh); and
- 0 - Banks 2 and 3 are active (memory locations 100h-1FFh).
- RP1,RP0 - Bits are used for selecting register bank at direct addressing.
RP1 |
RP0 |
ACTIVE BANK |
0 |
0 |
Bank0 |
0 |
1 |
Bank1 |
1 |
0 |
Bank2 |
1 |
1 |
Bank3 |
- TO - Time-out bit.
- 1 - After power-on, the execution of the
CLRWDT
instruction which resets the watch-dog timer or the SLEEP instruction which sets the microcontroller to the low-consumption mode.
- 0 - After the watch-dog timer time-out occurs.
- PD - Power-down bit.
- 1 - After power-on or the execution of the
CLRWDT
instruction which resets the watch-dog timer.
- 0 - After the execution of the
SLEEP
instruction which sets the microcontroller to the low-consumption mode.
- Z - Zero bit
- 1 - The result of an arithmetic or logic operation is zero.
- 0 - The result of an arithmetic or logic operation is different than zero.
- DC - Digit carry/borrow bit is changed during addition and subtraction if an ‘overflow’ or a ‘borrow’ of the result occurs.
- 1 - A carry-out from the 4th low-order bit of the result has occurred.
- 0 - No carry-out from the 4th low-order bit of the result has occurred.
- C - Carry/Borrow bit is changed during addition and subtraction if an ‘overflow’ or a ‘borrow’ of the result occurs, i.e. if the result is greater than 255 or less than 0.
- 1 - A carry-out from the most significant bit (MSB) of the result has occurred.
- 0 - No carry-out from the most significant bit (MSB) of the result has occurred.
OPTION_REG Register
Legend: R/W - Readable/Writable Bit, (1) After reset, bit is set
The OPTION_REG register contains various control bits which are used for configuring Timer0/WDT prescaler, Timer0, external interrupt and pull-ups on PORTB.
- RBPU - Port B Pull up Enable bit
- 1 - PORTB pull-ups are disabled.
- 0 - PORTB pull-ups are enabled.
- INTEDG - Interrupt Edge Select bit
- 1 - Interrupt on rising edge of the RB0/INT pin.
- 0 - Interrupt on falling edge of the RB0/INT pin.
- T0CS - TMR0 Clock Source Select bit.
- 1 - Transition on the T0CKI pin.
- 0 - Internal instruction cycle clock (Fosc/4).
- T0SE - TMR0 Source Edge Select bit selects pulse edge (rising or falling) counted by the Timer0 through the RA4/T0CKI pin.
- 1 - Increment on high-to-low transition on the TOCKI pin.
- 0 - Increment on high-to-low transition on the TOCKI pin.
- PSA - Prescaler Assignment bit assigns the prescaler (only one available) to the timer or watchdog timer.
- 1 - Prescaler is assigned to the WDT.
- 0 - Prescaler is assigned to the Timer0.
PS2, PS1, PS0 Prescaler Rate Select bits
A prescaler rate is selected by combining these bits. As shown in the table below, the prescaler rate depends on whether it is assigned to the Timer0 or watch-dog timer (WDT).
PS2 |
PS1 |
PS0 |
TMR0 |
WDT |
0 |
0 |
0 |
1:2 |
1:1 |
0 |
0 |
1 |
1:4 |
1:2 |
0 |
1 |
0 |
1:8 |
1:4 |
0 |
1 |
1 |
1:16 |
1:8 |
1 |
0 |
1 |
1:64 |
1:32 |
1 |
1 |
0 |
1:128 |
1:64 |
1 |
1 |
1 |
1:256 |
1:128 |
In order to achieve 1:1 prescaler rate when the Timer0 counts up pulses, the prescaler should be assigned to the WDT. As a result, the Timer0 does not use the prescaler, but directly counts pulses generated by the oscillator, which actually was the objective.
Let's do it in mikroBasic...
' If the CLRWDT command is not executed, WDT will reset the microcontroller every 32.768 uS
' (f=4 MHz)
OPTION_REG = %00001111 ' Prescaler is assigned to WDT (1:128)
asm
CLRWDT ' Assembly command to reset WDT
end asm
... ' Time between these two CLRWDT commands must not exceed 32.768 microseconds (128x256)
CLRWDT ' Assembly command to reset WDT
...
... ' Time between these two CLRWDT commands must not exceed 32.768 microseconds (128x256)
CLRWDT ' Assembly command to reset WDT
...
INTERRUPT SYSTEM REGISTERS
A reception of an interrupt request doesn’t mean that it will automatically occur, because it must also be enabled by the user (from within the program). For this reason, there are special bits used to enable or disable interrupts. It is easy to recognize them by letters IE (stands for Interrupt Enable) contained in their names. Besides, each interrupt is associated with another bit called a flag which indicates that an interrupt request has arrived regardless of whether it is enabled or not. Likewise, their names are followed by IF (Interrupt Flag).
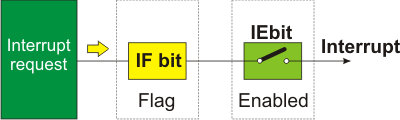
As you can see, everything is based on a simple and efficient principle. When an interrupt request arrives, the flag bit will be set first. If the appropriate IE bit is not set (0), the interrupt condition will be completely ignored. Otherwise, an interrupt occurs. If several interrupt sources are enabled, it is necessary to detect the active one before an interrupt routine starts execution. Source detection is performed by checking flag bits.
It is important to know that flag bits are not automatically cleared, but by software while the interrupt routine execution is under way. If we neglect this detail, another interrupt will occur immediately after returning to the main program, even though there are no more requests for its execution. Simply put, the flag, as well as the IE bit, remain set.
All interrupt sources typical of the PIC16F887 microcontroller are shown on the next page. Note several things:
The GIE bit enables all unmasked interrupts and disables all interrupts simultaneously.
The PEIE bit enables all unmasked peripheral interrupts and disables all peripheral interrupts. This doesn’t refer to Timer0 and PORTB interrupt sources.
To enable an interrupt to occur by changing the PORTB logic state, it is necessary to enable it for each bit separately. In this case, bits of the IOCB register act as control IE bits.
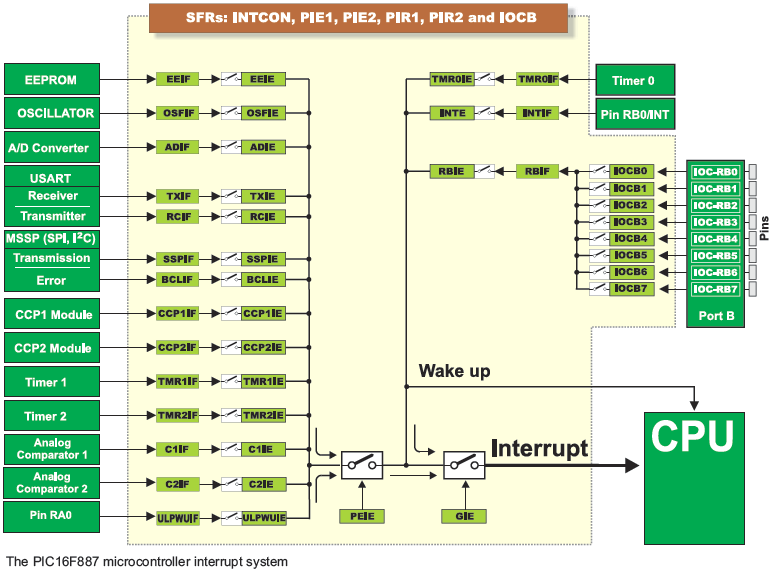
INTCON Register
The INTCON register includes various enable and flag bits for TMR0 register overflow, PORTB change and external INT pin interrupts.
Legend: R/W - Readable/Writable Bit, (0) After reset, bit is cleared, (X) After reset, bit is unknown
- GIE - Global Interrupt Enable bit - controls all possible interrupt sources simultaneously.
- 1 - All unmasked interrupts enabled.
- 0 - All interrupts disabled.
- PEIE - Peripheral Interrupt Enable bit acts similar to the GIE bit, but controls interrupts enabled by peripheral modules. Hence, it has no impact on interrupts triggered by Timer0 or by changing the state of port PORTB or pin RB0/INT.
- 1 - All unmasked peripheral interrupts enabled.
- 0 - All peripheral interrupts disabled.
- T0IE - TMR0 Overflow Interrupt Enable bit controls interrupts triggered by the TMR0 overflow.
- 1 - TMR0 overflow interrupt enabled.
- 0 - TMR0 overflow interrupt disabled.
- INTE - RB0/INT External Interrupt Enable bit controls interrupt caused by changing the logic state of the RB0/INT input pin (external interrupt).
- 1 - INT external interrupt enabled.
- 0 - INT external interrupt disabled.
- RBIE - RB Port Change Interrupt Enable bit. When configured as inputs, PORTB pins may cause an interrupt by changing their logic state (no matter whether it is high-to-low transition or vice versa, the fact that their logic state is changed only matters). The RBIE bit determines whether an interrupt is to occur or not.
- 1 - PORTB change interrupt enabled.
- 0 - PORTB change interrupt disabled.
- T0IF - TMR0 Overflow Interrupt Flag bit registers the Timer0 register overflow, when counting starts at zero.
- 1 - TMR0 register has overflowed (bit must be cleared from within the software).
- 0 - TMR0 register has not overflowed.
- INTF - RB0/INT External Interrupt Flag bit registers the change of the RB0/INT pin logic state.
- 1 - INT external interrupt has occurred (must be cleared from within the software).
- 0 - INT external interrupt has not occurred.
- RBIF - RB Port Change Interrupt Flag bit registers any change of the logic state of some PORTB input pins.
- 1 - At least one of the PORTB general purpose I/O pins has changed its logic state. After port PORTB read is complete, the RBIF bit must be cleared from within the software.
- 0 - None of the PORTB general purpose I/O pins has changed its logic state.
Let's do it in mikroBasic...
' The PORTB.4 pin is configured as an input sensitive to logic state change
ANSEL, ANSELH = 0 ' All I/O pins are configured as digital
PORTB = 0 ' All PORTB pins are cleared
TRISB = %00010000 ' All PORTB pins except PORTB.4 are configured as outputs
INTCON.RBIE = 1 ' Interrupts on PORTB change are enabled
IOCB.IOCB4 = 1 ' Interrupt on PORTB pin4 change is enabled
INTCON.GIE = 1 ' Global interrupt is enabled
...
...
'From this point, any change of the PORTB.4 pin logic state will cause an interrupt
PIE1 Register
The PIE1 register contains peripheral interrupt enable bits.
Legend: (-) Unimplemented bit, (R/W) - Readable/Writable Bit, (0) After reset, bit is cleared
- ADIE - A/D Converter Interrupt Enable bit.
- 1 - ADC interrupt enabled.
- 0 - ADC interrupt disabled.
- RCIE - EUSART Receive Interrupt Enable bit.
- 1 - Enables the EUSART receive interrupt.
- 0 - Disables the EUSART receive interrupt.
- TXIE - EUSART Transmit Interrupt Enable bit.
- 1 - EUSART receive interrupt enabled.
- 0 - EUSART receive interrupt disabled.
- SSPIE - Master Synchronous Serial Port (MSSP) Interrupt Enable bit - enables an interrupt request to be generated every time a data transfer via synchronous serial communication module (SPI or I2C mode) is complete.
- 1 - MSSP interrupt enabled.
- 0 - MSSP interrupt disabled.
- CCP1IE - CCP1 Interrupt Enable bit enables an interrupt request to be generated in CCP1 module used for PWM signal processing.
- 1 - CCP1 interrupt enabled.
- 0 - CCP1 interrupt disabled.
- TMR2IE - TMR2 to PR2 Match Interrupt Enable bit
- 1 - TMR2 to PR2 match interrupt enabled.
- 0 - TMR2 to PR2 match interrupt disabled.
- TMR1IE - TMR1 Overflow Interrupt Enable bit enables an interrupt request to be generated upon each TMR1 timer register overflow, i.e. when the counting starts from zero.
- 1 - TMR1 overflow interrupt enabled.
- 0 - TMR1 overflow interrupt disabled.
Let's do it in mikroBasic...
'Each Timer1 register (TMR1H and TMR1L) overflow causes an interrupt to occur. In every
'interrupt rutine, variable cnt will be incremented by 1.
dim unsigned short cnt ' Define variable cnt
sub procedure interrupt
cnt = cnt + 1 ' Interrupt causes cnt to be incremented by 1
PIR1.TMR1IF = 0 ' Reset bit TMR1IF
TMR1H = 0x80 ' TMR1H and TMR1L timer registers return
TMR1L = 0x00 ' their initial values
end sub
main:
ANSEL, ANSELH = 0 ' All I/O pins are configured as digital
T1CON = 1 ' Turn on Timer1
PIR1.TMR1IF = 0 ' Reset the TMR1IF bit
TMR1H = 0x80 ' Set initial value for Timer1
TMR1L = 0x00
PIE1.TMR1IE = 1 ' Enable an interrupt on overflow
cnt = 0 ' Reset variable cnt
INTCON = 0xC0 ' Enable interrupt (bits GIE and PEIE)
...
PIE2 Register
The PIE2 register also contains various interrupt enable bits.
Legend: (-) Unimplemented bit, (R/W) - Readable/Writable Bit, (0) After reset, bit is cleared
- OSFIE - Oscillator Fail Interrupt Enable bit.
- 1 - Oscillator fail interrupt enabled.
- 0 - Oscillator fail interrupt disabled.
- C2IE - Comparator C2 Interrupt Enable bit.
- 1 - Comparator C2 interrupt enabled.
- 0 - Comparator C2 interrupt disabled.
- C1IE - Comparator C1 Interrupt Enable bit.
- 1 - Comparator C1 interrupt enabled.
- 0 - Comparator C1 interrupt disabled.
- EEIE - EEPROM Write Operation Interrupt Enable bit.
- 1 - EEPROM write operation interrupt enabled.
- 0 - EEPROM write operation interrupt disabled.
- BCLIE - Bus Collision Interrupt Enable bit.
- 1 - Bus collision interrupt enabled.
- 0 - Bus collision interrupt disabled.
- ULPWUIE - Ultra Low-Power Wake-up Interrupt Enable bit.
- 1 - Ultra low-power wake-up interrupt enabled.
- 0 - Ultra low-power wake-up interrupt disabled.
- CCP2IE - CCP2 Interrupt Enable bit.
- 1 - CCP2 interrupt enabled.
- 0 - CCP2 interrupt disabled.
Let's do it in mikroBasic...
' Comparator C2 is configured to use pins RA0 and RA2 as inputs. Every change on
' the comparator's output will cause the PORTB.1 output pin to change its logic
' state in interrupt routine.
sub procedure interrupt
PORTB.F1 = not PORTB.F1 ' Interrupt will invert logic state of the PORTB.1 pin
PIR2.C2IF = 0 ' Interrupt flag bit C2IF is cleared
end sub
main:
TRISB = 0 ' All PORTB pins are configured as outputs
PORTB.1 = 1 ' Pin PORTB.1 is set
ANSEL = %00000101 ' RA0/C12IN0- and RA2/C2IN+ pins are analog inputs
ANSELH = 0 ' All other I/O pins are configured as digital
CM2CON0.C2CH0 = 0 ' The RA0 pin is selected to be C2 inverting input
CM2CON0.C2CH1 = 0
PIE2.C2IE = 1 ' Enables comparator C2 interrupt
INTCON.GIE = 1 ' Global interrupt is enabled
CM2CON0.C2ON = 1 ' Comparator C2 is enabled
...
...
PIR1 Register
The PIR1 register contains the interrupt flag bits.
Legend: (-) Unimplemented bit, (R/W) - Readable/Writable Bit, (R) - Readable Bit, (0) After reset, bit is cleared
- ADIF - A/D Converter Interrupt Flag bit.
- 1 - A/D conversion is completed (the bit must be cleared from within the software).
- 0 - A/D conversion is not completed or has not started.
- RCIF - EUSART Receive Interrupt Flag bit.
- 1 - EUSART receive buffer is full. The bit is cleared by reading the RCREG register.
- 0 - EUSART receive buffer is not full.
- TXIF - EUSART Transmit Interrupt Flag bit.
- 1 - EUSART transmit buffer is empty. The bit is cleared on any write to the TXREG register.
- 0 - EUSART transmit buffer is full.
- SSPIF - Master Synchronous Serial Port (MSSP) Interrupt Flag bit.
- 1 - All MSSP interrupt conditions during data transmit/receive are met. They differ depending on MSSP operating mode (SPI or I2C). This bit must be cleared from within the software before exiting the interrupt service routine.
- 0 - No MSSP interrupt condition met.
- CCP1IF - CCP1 Interrupt Flag bit.
- 1 - CCP1 interrupt condition is met (CCP1 is a unit for capturing, comparing and generating PWM signal). Operating mode indicates whether capture or compare match has occurred. In both cases, the bit must be cleared from within the software. This bit is not used in PWM mode.
- 0 - No CCP1 interrupt condition met.
- TMR2IF - Timer2 to PR2 Interrupt Flag bit
- 1 - TMR2 (8-bit register) to PR2 match has occurred. This bit must be cleared from within the software before exiting the interrupt service routine.
- 0 - No TMR2 to PR2 match has occurred.
- TMR1IF - Timer1 Overflow Interrupt Flag bit
- 1 - TMR1 register has overflowed. The bit must be cleared from within the software.
- 0 - TMR1 register has not overflowed.
PIR2 Register
The PIR2 register contains interrupt flag bits.
Legend: (-) Unimplemented bit, (R/W) - Readable/Writable Bit, (0) After reset, bit is cleared
- OSFIF - Oscillator Fail Interrupt Flag bit.
- 1 - Quartz oscillator failed and clock input has turned into internal oscillator INTOSC. This bit must be cleared from within the software.
- 0 - Quartz oscillator operates normally.
- C2IF - Comparator C2 Interrupt Flag bit.
- 1 - Comparator C2 output has changed (bit C2OUT). This bit must be cleared from within the software.
- 0 - Comparator C2 output has not changed.
- C1IF - Comparator C1 Interrupt Flag bit.
- 1 - Comparator C1 output has changed (bit C1OUT). This bit must be cleared from within the software.
- 0 - Comparator C1 output has not changed.
- EEIF - EE Write Operation Interrupt Flag bit.
- 1 - EEPROM write complete. This bit must be cleared from within the software.
- 0 - EEPROM write is not complete or has not started yet.
- BCLIF - Bus Collision Interrupt Flag bit.
- 1 - A bus collision has occurred in the MSSP when set to operate in I2C master mode. This bit must be cleared from within the software.
- 0 - No bus collision has occurred.
- ULPWUIF - Ultra Low-power Wake-up Interrupt Flag bit.
- 1 - Wake-up condition has occurred. This bit must be cleared from within the software.
- 0 - No wake-up condition has occurred.
- CCP2IF - CCP2 Interrupt Flag bit.
- 1 - CCP2 interrupt condition is met (unit for capturing, comparing and generating PWM signal). Operating mode indicates whether capture or compare match has occurred. In both cases, the bit must be cleared from within the software. This bit is not used in PWM mode.
- 0 - No CCP2 interrupt condition met.
Let's do it in mikroBasic...
' Module ULPWU activation sequence
main:
PORTA.0 = 1 ' PORTA.0 pin is set
ANSEL,ANSELH = 0 ' All I/O pins are configured as digital
TRISA = 0 ' PORTA pins are configured as outputs
Delay_ms(1) ' Charge capacitor
PIR2.ULPWUIF = 0 ' Clear flag
PCON.ULPWUE = 1 ' Enable ULP Wake-up
TRISA.0 = 1 ' PORTA.0 is configured as an input
PIE2.ULPWUIE = 1 ' Enable interrupt
INTCON.GIE = 1 ' Enable all unmasked interrupts
INTCON.PEIE = 1 ' Enable peripheral interrupt
asm ' Asm instruction
SLEEP ' Go to sleep mode
...
PCON register
The PCON register contains only two flag bits used to differentiate between power-on reset, brown-out reset, watchdog timer reset and external reset over the MCLR pin.
Legend: (-) Unimplemented bit, (R/W) - Readable/Writable Bit, (1) - After reset, bit is set, (0) After reset, bit is cleared
- ULPWUE - Ultra Low-Power Wake-up Enable bit
- 1 - Ultra low-power wake-up enabled.
- 0 - Ultra low-power wake-up disabled.
- SBOREN - Software BOR Enable bit
- 1 - Brown-out reset enabled.
- 0 - Brown-out reset disabled.
- POR - Power-on Reset Status bit
- 1 - No power-on reset has occurred.
- 0 - Power-on reset has occurred. This bit must be set from within the software after a power-on reset occurs.
- BOR - Brown-out Reset Status bit
- 1 - No brown-out reset has occurred.
- 0 - Brown-out reset has occurred. This bit must be set from within the software after a brown-out reset occurs.
PCL AND PCLATH REGISTERS
The size of the PIC16F887 program memory is 8K and provides 8192 locations for program storing. Consequently, the program counter (PC) must be 13 bits wide (213 = 8192). For the microcontroller to access some program memory location during the operation, its address must be available through SFRs. Since all SFRs are 8-bit wide, the program counter is created by combining two single registers. The low byte (8 bits) of the program counter occupies the PCL register, whereas the high byte (5 bits) occupies the PCLATH register. If the program execution doesn’t affect the program counter, the value of this register (PCL and PCLATH) is automatically and constantly incremented +1, +1, +1, +1... In this way, the program is executed as it is writteninstruction by instruction, followed by constant address increment. If the program counter is changed from within the software, then you should take care of the following:

If the program counter is changed from within the software, then there are several things that should be kept in mind in order to avoid problems:
- Eight lower bits of the PCL register (the low byte) are both readable and writable, whereas five upper bits of the PCLATH register are write-only.
- The PC register is cleared on any reset.
- In assembly language, the value of the program counter is marked as PCL and refers to 8 lower bits only. Be careful when using the 'ADDWF PCL' assembly instruction. This is a jump instruction, the destination of which is determined by adding some num ber to the current address. It is commonly used for jumping into a look-up table or pro gram branch table to read them. A problem arises if such addition causes the PCLATH register to be modified. Execution of any instruction upon the PCL register simultane ously causes the Program Counter bits to be replaced by the contents of both PCLATH and PCL register. However, the PCL register has access to 8 lower bits of the instruction result only so that the following jump will be incorrect. The problem is solved by setting such instructions to program addresses ending by xx00h. This enables the program to jump up to 255 locations. If longer jumps are executed by this instruction, the PCLATH register must be incremented by 1 for each PCL register overflow.
- On a subroutine call or jump execution (assembly instructions
CALL
and GOTO
),the microcontroller is capable of providing 11-bit address only. Similar to RAM, which is divided in ‘banks’, ROM is divided in four ‘pages’ of 2K each. Such instructions are properly executed within these boundaries. Simply put, since the processor deals with 11-bit address, it is capable of addressing any location within 2KB.
Figure below illustrates a jump to the subroutine PP1. But if the start of subroutine or jump address is not within the same page as the call destination, two ‘missing’ upper bits should be provided by performing the write operation to the PCLATH register. Figure below illustrates a jump to the subroutine PP2.
In both cases, when the subroutine reaches some of the following assembly instructions
RETURN
,
RETLW
or
RETFIE
(return to the main program), the microcontroller will simply proceed with the program execution from where it left off because the return address is pushed onto the stack which, as mentioned before, consists of 13-bit registers.
INDIRECT ADDRESSING
In addition to direct addressing, which is logical and self-obvious (it will do to specify the address of a register to read its contents), this microcontroller is also capable of performing indirect addressing by means of the INDF and FSR registers. It sometimes makes the process of writing a program easier. The whole procedure is enabled by the INDF register which is not a true one (it doesn’t physically exist). It is used to specify the register the address of which is stored in the FSR register. As a result, write to or read from the INDF register actually means write to or read from the register the address of which is stored in the FSR register. In other words, registers’ addresses are stored in the FSR register, whereas their contents are stored in the INDF register. Figure below illustrates the difference between direct and indirect addressing.
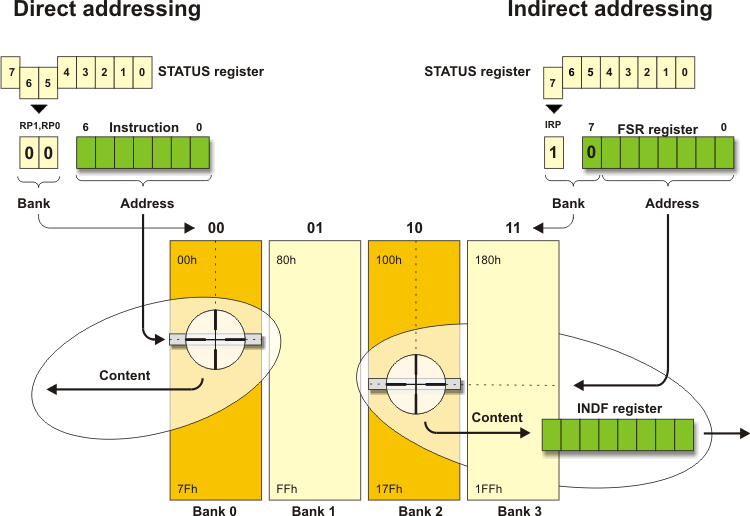
As can be seen, the ‘missing addressing bits’ issue is solved by a ‘borrow’ from another register. This time, it is the seventh bit, called the IRP bit, of the STATUS register.